22 Calling packages
Written by Mariam Walaa and last updated on 7 October 2021.
22.1 Introduction
In this lesson, you will learn how to:
- Load an R package with the correct syntax
- Understand R’s output when you call a package
- Understand what R does when multiple packages use the same function name
Prerequisite skills include:
- Installing packages
Highlights:
- An R package needs to be loaded in order to use its functions.
- An R package needs to be loaded using the appropriate syntax.
- R provides important details about a package when you load it.
- R has a way of handling packages using the same function name.
22.3 library()
The library()
function takes the following as arguments:
Argument | Parameter | Details |
---|---|---|
package* | package name | this takes package name in or not in quotations |
help | package name | this displays the help for the specified package |
logical.return | Boolean | this returns a Boolean on whether package is loaded |
quietly | Boolean | this suppresses confirmations if set to True |
verbose | Boolean | this suppresses diagnostics if set to False |
*This is a required argument.
Note that you if you want to load the help using library()
, you may need to pass the
‘help’ parameter alone without the others. If you would like more information about the
arguments, please visit the R documentation for this function
here.
22.4 Overview
Loading packages properly is a necessary aspect of running code in R. Loading a package is needed in order to be able to use functions in that package.
Here is what loading a package looks like.
Note that some functions are already provided in base R and do not belong to specific
packages. You will see in future tutorials that this is the case for the functions lm()
and summary()
.
Besides knowing how to load packages and which packages to load, it’s important to understand what R does when multiple packages use the same function name. This may be the cause of many errors you have in your code. There are two common scenarios that may lead to errors related to this issue in your code:
You don’t load a package you need to use a function, but the function name exists in base R, so R tries to use the base R function but gets into issues since you probably don’t have the appropriate parameters for that function.
You load the package you need to use a function, but you load another package after, and that package contains the same function name and masks* the initial package.
*When R masks a package with another package for a specific function, it is deciding to use the function belonging to the package that has masked the other package.
The best way to avoid running into this issue is to change the order in which you are
calling the packages so that the package whose functions you need is last, or to let R
know which package you want to use a function from. For example, if you want to use
select()
from dplyr and not some other package, you can specify dplyr::select()
.
Here is a visualization to help you understand when to use the different package-related
functions. Please note that it is a lot more common to use library()
over require()
.
You can use require()
if you want your code to continue running even if the package did
not load correctly. You can learn more about require()
in its documentation
here.
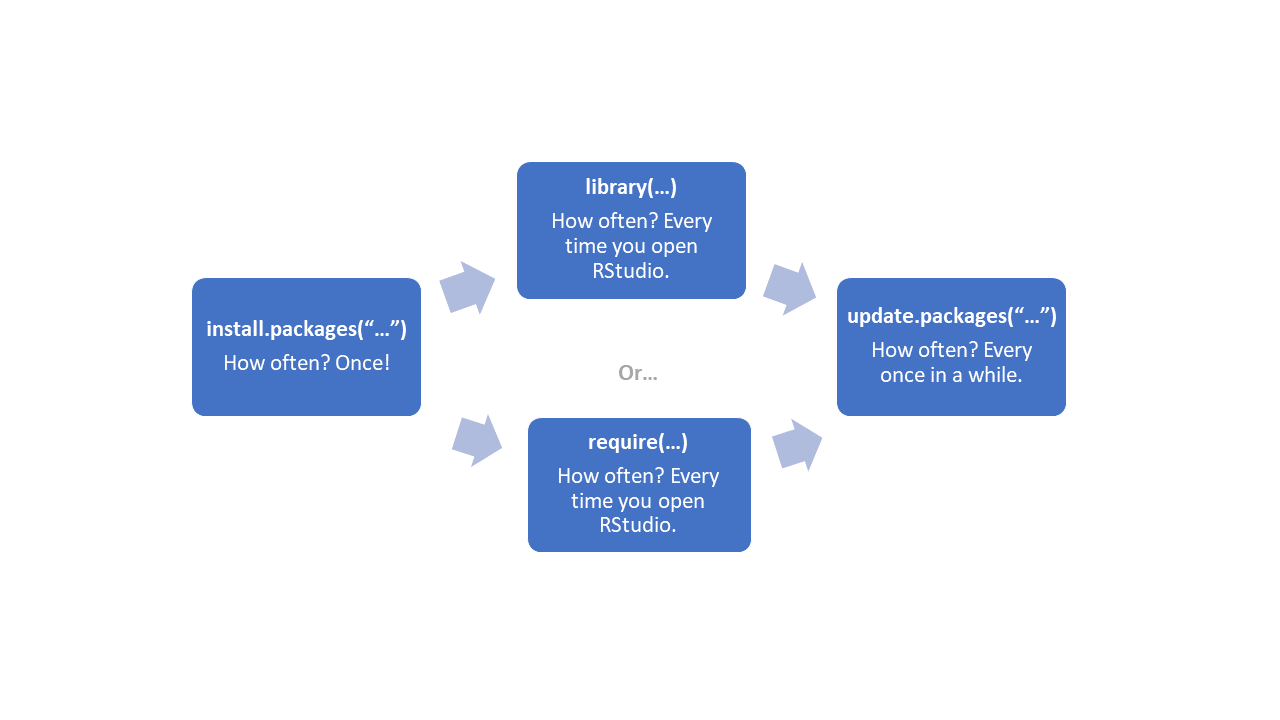
22.4.1 Citing R
When we use R in a formal setting (such as, when writing a paper), we will want to cite R.
This can be done with some guidance from the citation()
function.
citation()
#>
#> To cite R in publications use:
#>
#> R Core Team (2021). R: A language and environment
#> for statistical computing. R Foundation for
#> Statistical Computing, Vienna, Austria. URL
#> https://www.R-project.org/.
#>
#> A BibTeX entry for LaTeX users is
#>
#> @Manual{,
#> title = {R: A Language and Environment for Statistical Computing},
#> author = {{R Core Team}},
#> organization = {R Foundation for Statistical Computing},
#> address = {Vienna, Austria},
#> year = {2021},
#> url = {https://www.R-project.org/},
#> }
#>
#> We have invested a lot of time and effort in creating
#> R, please cite it when using it for data analysis.
#> See also 'citation("pkgname")' for citing R packages.
Please refer to the References and Bibtex section in the toolkit for more information on why it is important to cite R and R packages.
22.6 Exercises
22.6.4 Exercise 4
Select all the true statements about the library()
function and calling libraries.
22.7 Common Mistakes & Errors
Here are some common mistakes and errors you may come across:
- You try to load a package that has not been installed yet.
- You try to use a function from a package that has not been loaded yet.
- You try to load a package but you receive the following error message:
Error in library(...) : there is no package called ‘...’
. This is most likely due to a typo:- You have misspelled the package name (i.e. “tdyverse” instead of “tidyverse”)
- You have not used the correct casing (i.e. “Tidyverse” instead of “tidyverse”)
If you run into other issues while trying to load packages, try to restart R, re-install the package, and load the package again.
22.8 Next Steps
If you would like to learn more about functions for packages, here are some additional functions you may find helpful:
- You can run
search()
to see currently attached packages. - You can run
library()
to see currently installed packages. - You can run
library(help = 'package_name')
to see package help in RStudio.- This may be faster than searching it up and possibly more up-to-date with the most recent version of the package.
- You can run
detach("package:_")
with the package name to detach a package from session.
If you would like to read more about packages, here are some additional resources you may find helpful:
- Hands-On Programming with R
- CRAN: An Introduction to R